-
Flo zum Verfolgen des Zyklus
-
Flo zum Schwangerwerden
-
Flo für Schwangere
-
Anonymer Modus
-
Flo-Bewertungen
-
Flo Premium
-
Dein Zyklus
-
Gesundheit 360°
-
Schwanger werden
-
Schwangerschaft
-
Eine Mutter sein
-
Eisprungrechner
-
hCG-Rechner
-
Periodenrechner
-
SSW-Rechner
-
Geburtsterminrechner für IVF und Kryotransfer
-
Geburtstermin anhand von Ultraschall
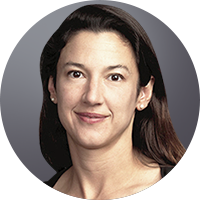
Dr. DeTata ist eine Geburtshelferin und Gynäkologin mit dem Schwerpunkt auf komplizierte Schwangerschaften.
Als klinische Assistenzprofessorin an der Stanford University leitet sie die Kurse in Geburtshilfe und Gynäkologie für die Studenten im 3. und 4. Studienjahr.
Sie hat sich im Laufe ihrer Karriere um Tausende Patienten gekümmert und Tausend Medizinstudenten unterrichtet. Sie hat national bei mehreren Konferenzen Vorträge gehalten und ist Redakteurin bei „The Clinical Teacher“. Sie ist zudem Gastdozentin an der University of Southern California’s School of Medicine.
Sie ist ein aktives Mitglied verschiedener professioneller Gesellschaften im Bereich Geburtshilfe, Gynäkologie und Ausbildung, darunter dem American College of Obstetrics and Gynecology, den American Professors of Gynecology and Obstetrics und Stanford’s Teaching and Learning Academy.
Dr. DeTata arbeitet gerne mit ihren Patienten zusammen, um ihnen zu helfen, Gesundheitsvorsorge zu verstehen, sie damit zu unterstützen und sie ihnen zu erleichtern. Sie hat erfolgreich vielen Patientinnen, die ein Kind möchten, bei zugrundeliegenden Krankheiten geholfen, so dass sie eine gesunde Schwangerschaft und ein gesundes Baby haben konnten. Sie glaubt, dass genaues Wissen Frauen die Macht gibt, ihre eigene Gesundheit zu kontrollieren. Sie fühlt sich geehrt, als medizinische Beraterin bei Flo dabeizusein.